In the world of programming, especially in object-oriented programming, there are several key principles and concepts that help developers create organized and maintainable code. One of these essential principles is encapsulation. Encapsulation in python is a fundamental concept that encourages the organization of data and functionality within classes and objects, and it is particularly relevant in Python, a versatile and object-oriented language.
What is Encapsulation in Python?
At its core, encapsulation is about ‘hiding the internal details of an object’ and providing a well-defined interface to interact with it. It’s like putting your object’s data and functionality in a protective capsule, allowing controlled access. This concept is crucial for maintaining the integrity of objects and ensuring that they are used correctly in a program.
In Python, you can achieve encapsulation by using access control modifiers, naming conventions, and properties.
Access Control Modifiers
Python provides three access control modifiers to indicate the visibility of attributes and methods within a class:
- Public: Attributes and methods with no access modifier are considered public. They can be accessed from both inside and outside the class.
- Protected: In Python, attributes and methods with a single leading underscore (e.g., `_variable` or `_method()`) are considered protected. While they can still be accessed from outside the class, it’s a convention indicating that they should be treated as non-public parts. These elements are meant for internal use within the class.
- Private: Attributes and methods with a double leading underscore (e.g., `__variable` or `__method()`) are considered private. They are name-mangled to include the class name, making them more challenging to access from outside the class. While not entirely private, they are intended for internal use within the class.
Name Mangling
Private attributes and methods in Python are not entirely hidden. They are transformed by the interpreter into names that include the class name. This is called name mangling. It’s a mechanism that makes it more challenging to access private members from outside the class, as the name is altered.
Example
For example, if a class `MyClass` has a private attribute `__my_private_var`, it will be transformed into `_MyClass__my_private_var`. This effectively prevents accidental or unwanted access from external code.
Properties
In addition to access control modifiers, Python offers a powerful feature for encapsulation called properties. Properties allow you to control the access to attributes by defining custom getter and setter methods. This means that you can enforce validation, computation, or any other behavior when getting or setting an attribute.
Here’s an example of a Python class using properties for encapsulation:
In this example, the `radius` attribute is protected using the single underscore naming convention. We also define a property for `radius`, allowing us to control how it’s accessed and modified. We enforce the rule that the radius must be positive, ensuring data integrity.
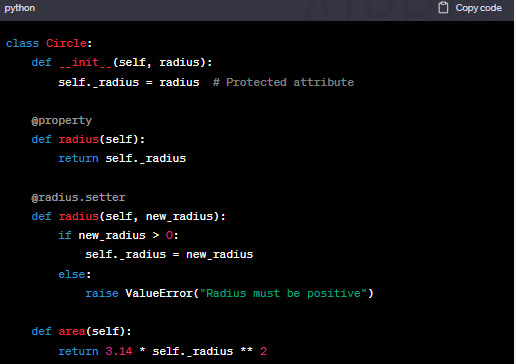
Benefits of Encapsulation
Encapsulation provides several important benefits:
- Data Integrity: By controlling access to attributes and methods, you can ensure that your object’s data remains in a valid state.
- Abstraction: Encapsulation allows you to abstract the internal details of an object. This makes it easier to use and understand objects by providing a clear and well-defined interface.
- Flexibility: You can modify the internal representation of an object without affecting the code that uses it. This is known as encapsulating the implementation details.
- Security: It helps protect sensitive data from unauthorized access and manipulation.
- Debugging and Maintenance: When issues arise, encapsulation makes it easier to locate and fix problems, as the scope of investigation is limited to the object’s methods.
Best Practices for Encapsulation
To make the most out of encapsulation in Python, consider the following best practices:
- Use access control modifiers (public, protected, private) and name mangling to indicate the visibility and importance of attributes and methods.
- Use properties when you need to control how attributes are accessed or modified.
- Document your classes and their public interfaces to make it clear to other developers how to use your code.
- Follow naming conventions. By convention, protected attributes and methods use a single leading underscore, and private attributes and methods use a double leading underscore.
- Avoid direct access to protected and private attributes from outside the class whenever possible. Instead, provide public methods to access or modify these attributes if necessary.
Conclusion
Encapsulation in Python is a crucial concept and object-oriented programming in general. It allows you to create well-structured, maintainable, and secure code by controlling access to the internal details of your objects. By using access control modifiers, name mangling, and properties, you can strike a balance between data protection and accessibility, improving the robustness and reliability of your Python programs.